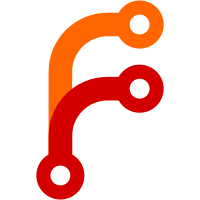
We want Vault to perform token reviews with Kubernetes even if we are using an external Vault. We need to create the ServiceAccount, Secret and ClusterRoleBinding with the system:auth-delegator role to enable delegated authentication and authorization checks [1]. These SA and RBAC objects are created when we deploy the Vault server. In order to enable the creation of these objects when using an external Vault, we remove the condition on external mode. User might want to provide a sensible name (in global.serviceAccount.name) to the service account such as: vault-auth. refs #376 [1] https://www.vaultproject.io/docs/auth/kubernetes#configuring-kubernetes
72 lines
2.4 KiB
Bash
Executable file
72 lines
2.4 KiB
Bash
Executable file
#!/usr/bin/env bats
|
|
|
|
load _helpers
|
|
|
|
@test "server/ClusterRoleBinding: enabled by default" {
|
|
cd `chart_dir`
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'server.dev.enabled=true' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "true" ]
|
|
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'server.ha.enabled=true' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "true" ]
|
|
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "true" ]
|
|
}
|
|
|
|
@test "server/ClusterRoleBinding: disable with global.enabled" {
|
|
cd `chart_dir`
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'global.enabled=false' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "false" ]
|
|
}
|
|
|
|
@test "server/ClusterRoleBinding: can disable with server.authDelegator" {
|
|
cd `chart_dir`
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'server.authDelegator.enabled=false' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "false" ]
|
|
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'server.authDelegator.enabled=false' \
|
|
--set 'server.ha.enabled=true' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "false" ]
|
|
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'server.authDelegator.enabled=false' \
|
|
--set 'server.dev.enabled=true' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "false" ]
|
|
}
|
|
|
|
@test "server/ClusterRoleBinding: also deploy with injector.externalVaultAddr" {
|
|
cd `chart_dir`
|
|
local actual=$( (helm template \
|
|
--show-only templates/server-clusterrolebinding.yaml \
|
|
--set 'injector.externalVaultAddr=http://vault-outside' \
|
|
. || echo "---") | tee /dev/stderr |
|
|
yq 'length > 0' | tee /dev/stderr)
|
|
[ "${actual}" = "true" ]
|
|
}
|